“Delegate functions” is a way to support treating lambda expressions as functions. Consider the following example:
static void Main(string[] args)
{
DelFunc.F1 add = (int a, int b) => a + b;
var add2 = add.Apply(a: 2);
var twoPlusThree = add2.Invoke(b: 3);
}
The DelFunc.F1 type, will be generated by the DIVEX upon save. Now, add is a function as far as DIVEX is concerned. In the example above, Apply is used to partially apply the add function.
These auto-generated DelFunc types should only be used once per generated type. Therefore, the following is not valid usage:
DelFunc.F1 add = (int a, int b) => a + b;
DelFunc.F1 subtract = (int a, int b) => a - b;
DIVEX will refuse to generate the DelFunc.F1 type in this case. The solution is to use something other than F1:
DelFunc.F1 add = (int a, int b) => a + b;
DelFunc.F2 subtract = (int a, int b) => a - b;
The experience of dealing with DelFunc is more pleasant than this because of some Visual Studio editor features that DIVEX brings. For example, you can write delfunc and IntelliSense will replace it with an appropriate DelFunc.*:
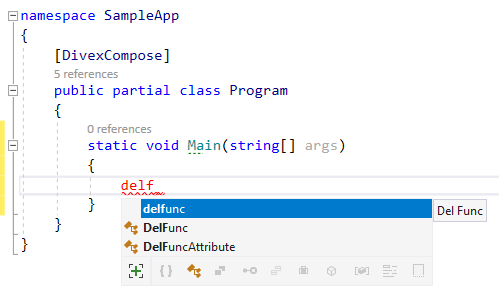
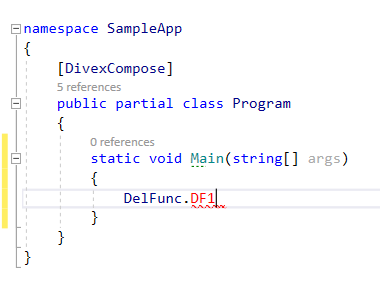
In the above figures, IntelliSense inserted the code DelFunc.DF1 as a result of me choosing the delfunc IntelliSense completion item. Now, once I save, Visual Studio displays func instead of DelFunc.DF1 to make it look better:
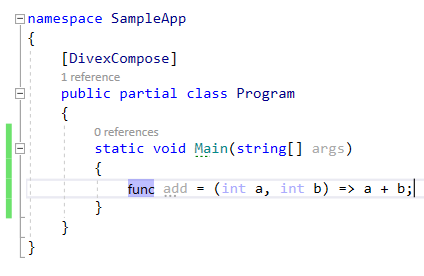
The next time the delfunc completion item is used via IntelliSense, DIVEX will use DF2 instead of DF1.
Delegate functions can also be used inline like this:
static void Main(string[] args)
{
DelFunc.DF1 writeInteger = (int i) => Console.WriteLine(i);
var writeLengthOfString = writeInteger
.Replace(i: (DelFunc.DF2) ((string str) => str.Length));
}
Basically, a lambda was used as a function by casting it to a DelFunc (DelFunc.DF2 in this case). Again, the experience is more pleasant in Visual Studio. The following GIF shows the experience of writing the above code in Visual Studio:
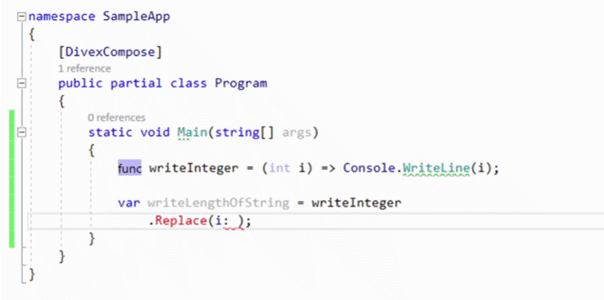